React 19: A Comprehensive Guide to the Latest Features
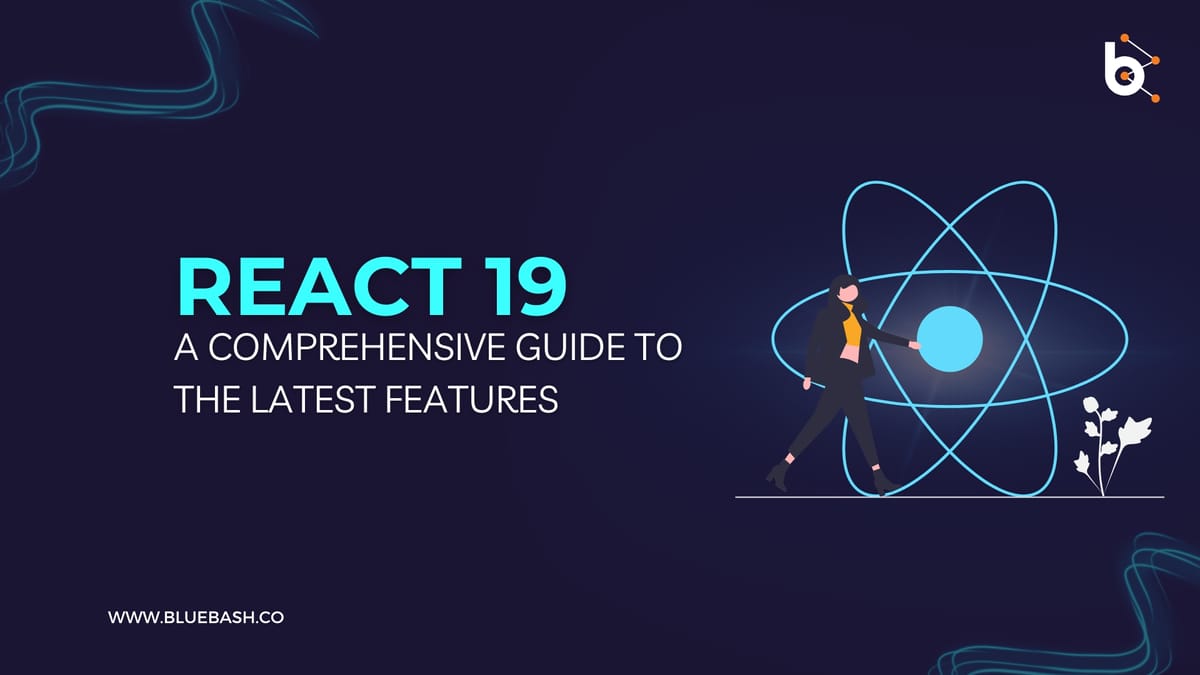
React is still at the forefront of web development because to its creative upgrades that improve developer experience and efficiency. The most recent version, React 19, offers a number of capabilities that are expected to completely change the way we develop online apps. We will examine the main components of React 19 in-depth and learn how they may change the way you write software.
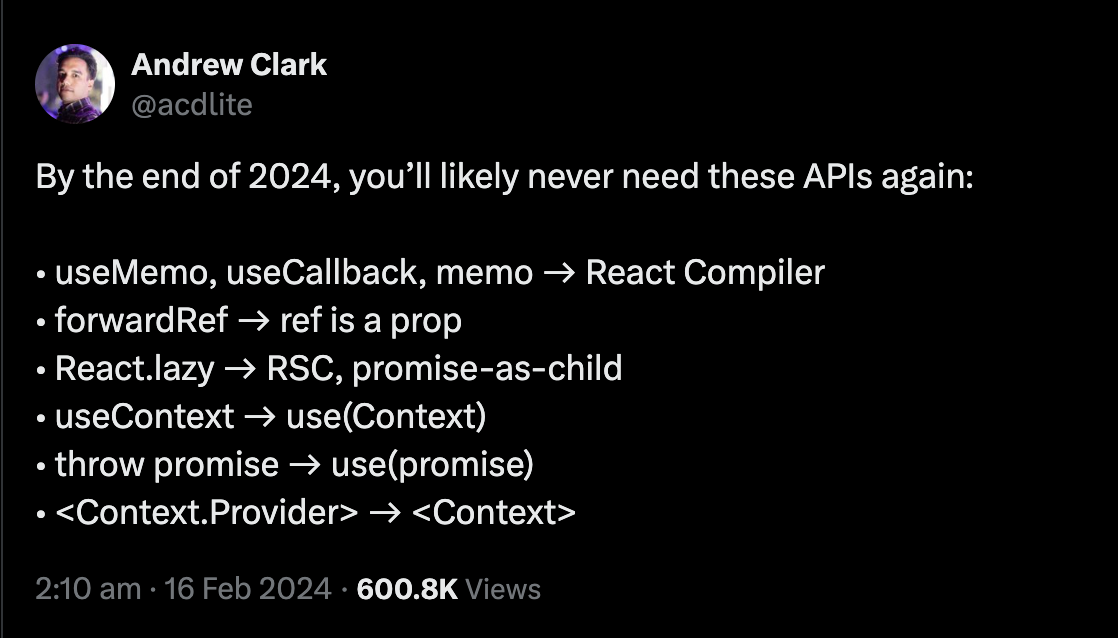
Key Enhancements :
1. Improved Concurrent Mode
The enhanced Concurrent Mode in React 19 is one of its most notable improvements. React can manage numerous tasks at once in this mode, which improves the responsiveness and efficiency of apps.
- Scheduler API & Enhanced Suspense for Data Fetching:
- Prioritized Rendering with
useTransition
:useTransition
allows us to defer the update of thesearchQuery
used by theItemList
component. This ensures that the input field remains responsive even while the item list is being updated.startTransition
is used to wrap the state update that triggers the background update. This indicates to React that this update is of lower priority.
- Fluid User Interactions with
useDeferredValue
:useDeferredValue
is employed to produce a postponed version of thesearchQuery
. This deferred value updates at a lower priority, ensuring that the input field remains responsive.- The
ItemList
component receives the deferred value, which means it only updates when there are available resources, avoiding any jank or lag in the input field.
- Suspense for Data Fetching:
- The
Suspense
component is used to handle the loading state of theItemList
. While the items are being fetched, a fallback message "Loading items..." is displayed. - This provides a seamless experience where the input remains interactive, and the user is informed that the item list is loading.
- The
2. React Server Components (RSC)
Developers may now create components that are rendered on the server and transmitted to the client thanks to React 19's expansion of Server Components. This feature minimizes the amount of JavaScript that must be run on the client side in an effort to improve performance.
Benefits:
- Reduced Client-Side Load: By rendering the
ServerComponent
on the server, the client handles less JavaScript, resulting in faster load times and better performance, especially on devices with limited processing power.. - SEO Improvements: The server-rendered HTML is complete and ready for indexing by search engines, improving the application's visibility and search engine ranking.
3. Improved State Management
In order to simplify state management, React 19 adds new hooks and utilities that reduce the need on external libraries and facilitate the handling of complex state scenarios.
Benefits:
- Simplified State Logic: More user-friendly methods for managing state changes and asynchronous operations are offered via new hooks like useTransition and useDeferredValue.
- Less Boilerplate: Codebases can be kept cleaner and easier to maintain when built-in solutions eliminate the requirement for large amounts of boilerplate code.
What’s New in React 19?
1. The React Compiler 🤖
- React code is transformed into standard JavaScript via the React Compiler, which could double performance. It's similar to adding more power to your code.
- Currently, React requires developers to manually optimize re-renders using
useMemo()
,useCallback()
, andmemo
APIs. This manual optimization, described by the React team as a "reasonable manual compromise," involves developers deciding when and how to update the UI based on state changes.However, recognizing the complexity and feedback from the community about the cumbersome nature of these manual optimizations, the React team introduced the "React Compiler." This new tool automates the management of re-renders, allowing React to determine the optimal times and methods for state updates and UI changes. - With the React Compiler, react developers no longer need to manually handle these optimizations. The need for
useMemo()
,useCallback()
, andmemo
is eliminated, as React now automatically manages re-renders, simplifying the development process and enhancing performance.
2. Actions API 💪
- The introduction of server components enables the execution of Actions on the server side. In JSX, within a
<form/>
element, the traditionalonSubmit
event can be replaced with theaction
attribute. This attribute's value will specify a method for submitting data, either on the client or server side. - Actions allow for the execution of both synchronous and asynchronous operations, simplifying the management of data submission and state updates. The objective is to streamline the process of working with forms and handling data.
- To see how this functions, let's examine an example:
const submitData = async (inputData) => {
const inputUser = {
username: inputData.get('username'),
email: inputData.get('email')
}
console.log(inputUser)
}
const Form = () => {
return (
<form action={submitData}>
<div>
<label>Name</label>
<input type="text" name='username'/>
</div>
<div>
<label>Name</label>
<input type="text" name="email" />
</div>
<button type='submit'>Submit</button>
</form>
);
}
export default Form;
The action in the server component of the given code snippet is submitData. The <Form>
component is a client-side component that utilizes submitData
as the action. It's important to note that submitData
will be executed on the server. The communication between the client-side (Form) and server-side (submitData) components is facilitated solely by the action attribute.
3. Server Components 🔥
- One of the most exciting developments in Next.js and React, if you haven't heard about server components yet, is on you.
React components have often operated on the client side in the past.
However, React is now introducing the innovative concept of server-side components.The concept of server components has been around for years, with Next.js leading the charge in making them production-ready. Beginning with Next.js 13, all components default to being server components. To specify that a component should run on the client side, you must use the "use client" directive. In React 19, server components will be natively integrated, providing numerous advantages: - SEO: Server-rendered components enhance search engine optimization by making content more accessible to web crawlers.
- Performance Boost: Server components improve initial page load times and overall performance, especially for applications with heavy content.
- Server-Side Execution: They allow code to be executed on the server, making tasks like API calls seamless and efficient.
These benefits highlight the transformative potential of server components in modern web development.
By default, all components in React are client-side. To make a component run on the server, you add the 'use server' directive at the beginning of the component. This converts the component into a "server component," which will only run on the server side and not on the client side.
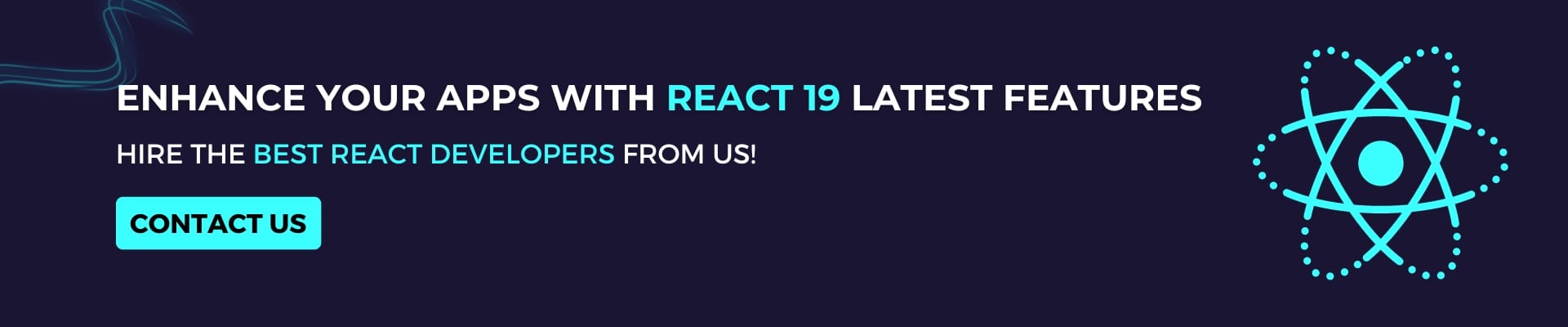
Here’s how you can use a server component:
You can import a server component, such as requestUsername
, into any React component within the same project. After importing, you can use "Actions" (details on this will follow) to perform specific tasks.This shift to server components marks a significant improvement in how React applications can be built and optimized, offering enhanced performance and SEO benefits out of the box.
'use server';
export default async function clientUsername(inputData) {
const username = inputData.get('username');
if (canRequest(username)) {
// ...
return 'successful';
}
return 'failed';
}
Currently, Next.js supports server-side components.With React 19, server component support will be integrated directly into React.
4. Document Metadata 🌇
- Elements such as "title," "meta tags," and "description" are essential for optimizing SEO and ensuring accessibility. In React, particularly with single-page applications, managing these elements across different routes can be challenging.
- Currently, developers frequently write custom code or use packages like react-helmet to manage route changes and update metadata. This process can be tedious and prone to errors, especially when handling SEO-critical elements like meta tags.
Before:
import React, { useEffect } from 'react';
const MetaManager = ({ pageTitle }) => {
useEffect(() => {
document.title = pageTitle;
const descriptionMetaTag = document.querySelector('meta[name="description"]');
if (descriptionMetaTag) {
descriptionMetaTag.setAttribute('content', 'New description');
}
}, [pageTitle]);
return null;
};
export default MetaManager;
In the code above, we have a component named HeadDocument
that updates the title and meta tags based on the provided props. This is done within the useEffect
hook, using JavaScript to modify the title and meta tags. The component updates whenever the route changes. However, this approach is not the most efficient or clean way to handle such updates.
After:
const LandingPage = () => {
return (
<>
<title></title>
<meta name="description" content="Freecode camp blogs" />
{/* Page content */}
</>
);
}
5. Enhanced Hooks & Asset Loading 💼
- In React, managing the loading experience and performance of applications, especially with images and other assets, is crucial.
- Typically, the browser first renders the view, followed by stylesheets, fonts, and images, leading to a flash of unstyled content before the styled view is displayed.To address this issue, developers often implement custom code to detect when assets are ready, ensuring that the view is shown only after everything has loaded.
- In React 19, images and other files will load in the background as users explore the current page. This enhancement aims to improve page load times and reduce waiting periods.
- Additionally, React introduces lifecycle Suspense for assets loading, including scripts, stylesheets, and fonts. This feature enables React to determine when content is ready to be displayed, eliminating any "unstyled" flickering.
- New Resource Loading APIs like preload and preinit offer greater control over when a resource should load and initialize.
- By enabling assets to load asynchronously in the background, React 19 minimizes waiting times and ensures uninterrupted interaction with the content. This optimization not only boosts the performance of React applications but also enhances the browsing experience for users.
6. New React Hooks 🪝🪝
React Hooks have emerged as one of the most beloved features in the library. Chances are, you've utilized React's built-in hooks extensively, and maybe you've even experimented with creating your own custom hooks. Hooks have gained widespread popularity to the extent that they've established themselves as a common programming pattern in React.
- In React 19, the way we use
useMemo
,forwardRef
,useEffect
, anduseContext
will change. This is mainly because a new hook,use
, will be introduced.
useMemo()
:
You won't need to use the useMemo()
hook after React19, as React Compiler will memoize by itself.
Before:
import React, { useState, useMemo } from 'react';
function ExampleComponent() {
const [inputValue, setInputValue] = useState('');
// Memoize the result of checking if the input value is empty
const isInputEmpty = useMemo(() => {
console.log('Checking if input is empty...');
return inputValue.trim() === '';
}, [inputValue]);
return (
<div>
<input
type="text"
value={inputValue}
onChange={(e) => setInputValue(e.target.value)}
placeholder="Type something..."
/>
<p>{isInputEmpty ? 'Input is empty' : 'Input is not empty'}</p>
{/* Additional paragraph to display the length of the input value */}
<p>Length of input: {inputValue.length}</p>
</div>
);
}
export default ExampleComponent;
After:
In the below example, you can see that after React19, we don't need to memo the values – React19 will do it by itself under the hood.
import React, { useState } from 'react';
function ExampleComponent() {
const [inputValue, setInputValue] = useState('');
const isInputEmpty = () => {
console.log('Checking if input is empty...');
return inputValue.trim() === '';
};
return (
<div>
<input
type="text"
value={inputValue}
onChange={(e) => setInputValue(e.target.value)}
placeholder="Type something..."
/>
{/* Ensure to call the function isInputEmpty */}
<p>{isInputEmpty() ? 'Input is empty' : 'Input is not empty'}</p>
</div>
);
}
export default ExampleComponent;
The newuse()
hook
React19 will introduce a new hook called use()
. This hook will simplify how we use promises, async code, and context.
syntax of hook:
const value = use(resource);
You can use the use
hook to make a fetch
request:
import { useState, useEffect } from "react";
const useFetchUsers = () => {
const [users, setUsers] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
const fetchUsers = async () => {
try {
const res = await fetch('https://jsonplaceholder.typicode.com/users');
const data = await res.json();
setUsers(data);
setLoading(false);
} catch (error) {
console.error('Error fetching users:', error);
setLoading(false);
}
};
fetchUsers();
// Clean-up function
return () => {
// Any clean-up code if needed
};
}, []);
return { users, loading };
};
const UsersItems = () => {
const { users, loading } = useFetchUsers();
if (loading) {
return <p>Loading...</p>;
}
return (
<ul>
{users.map((user) => (
<div key={user.id} className='bg-blue-50 shadow-md p-4 my-6 rounded-lg'>
<h2 className='text-xl font-bold'>{user.name}</h2>
<p>{user.email}</p>
</div>
))}
</ul>
);
};
export default UsersItems;
useFetchUsers
is a custom hook responsible for fetching users data asynchronously and managing the loading state.- Inside
UsersItems
, we useuseFetchUsers
to get the users data and loading state, and then render the UI accordingly. - While fetching data, it displays a loading message, and once the data is fetched, it renders the users' information.
In conclusion, React 18 is still a good option for reliable applications even though React 19 offers exciting new features. When selecting between these versions, keep your project's requirements and objectives in mind! 🚀
FAQ's
What are the major enhancements in React 19?
Improved Concurrent Mode: Allows React to manage multiple tasks simultaneously, enhancing app responsiveness and efficiency.
React Server Components (RSC): Enables components to be rendered on the server and sent to the client, reducing client-side load and improving performance.
Improved State Management: Introduces new hooks and utilities to simplify state management without relying on external libraries.
How does the enhanced Concurrent Mode in React 19 work?
Scheduler API & Enhanced Suspense: Helps prioritize rendering tasks and handle data fetching more efficiently.
useTransition Hook: Allows deferring updates to keep the UI responsive.
useDeferredValue Hook: Updates values at a lower priority, improving input responsiveness.
Suspense for Data Fetching: Displays a fallback message during data fetching, ensuring a seamless user experience.
What are React Server Components (RSC) and their benefits?
Reduced Client-Side Load: Server-rendered components decrease the amount of JavaScript executed on the client, leading to faster load times.
SEO Improvements: Server-rendered HTML improves search engine indexing and visibility.
What is the React Compiler and how does it improve performance?
Automatic Optimization: Eliminates the need for manual optimization with useMemo, useCallback, and memo APIs.
Enhanced Performance: Transforms React code into optimized JavaScript, potentially doubling performance.
What new hooks are introduced in React 19 and how do they change the development process?
use Hook: Simplifies handling promises, async code, and context.
Elimination of useMemo: React Compiler handles memoization automatically, removing the need for useMemo.
Can you give an example of how the new use hook works?
Syntax: const value = use(resource);
Example: Fetching data using use to handle asynchronous operations within React components.