PDF / CSV ChatBot with RAG Implementation (Langchain and Streamlit) - A step-by-step Guide
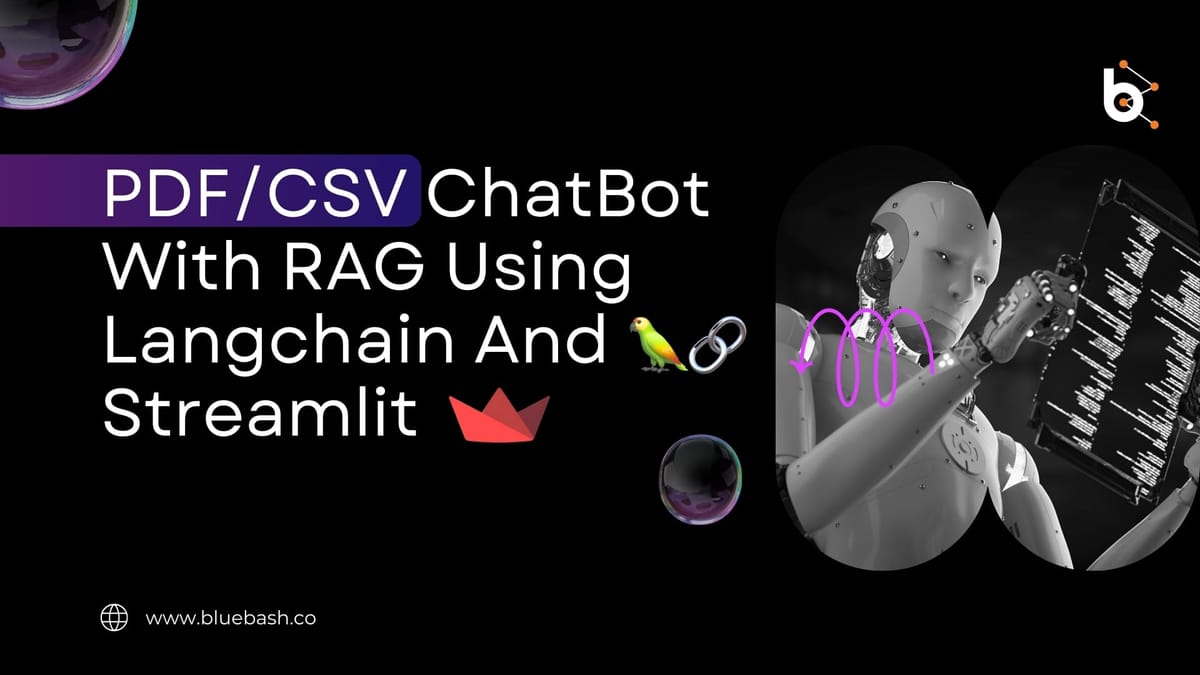
In the rapidly evolving landscape of artificial intelligence (AI) and machine learning (ML), Retrieval-Augmented Generation (RAG) stands out as a groundbreaking framework designed to enhance the capabilities of large language models (LLMs). By leveraging external knowledge bases, RAG significantly improves the accuracy and relevance of generated content, ensuring that the outputs are not only original but also grounded in the most current and authoritative sources available.
At its core, RAG acts as a bridge between the generative prowess of LLMs, such as the Generative Pre-trained Transformer models, and the vast stores of information contained within external databases. This synergy allows for the production of content that is both rich in quality and highly informative, addressing one of the key challenges faced by traditional LLMs: the reliance on static training data that quickly becomes outdated. By integrating Artificial intelligence solutions, RAG enhances the ability to generate up-to-date, contextually relevant content from dynamic information sources.
How RAG Enhances Accuracy and Transparency in NLP?
The significance of RAG in Artificial Intelligence, especially in NLP and content generation, lies in its ability to dynamically incorporate external data, enabling businesses to deliver precise, up-to-date information. This enhances user experiences with more accurate responses and broadens AI applications across various industries. RAG also introduces transparency by allowing users to trace information sources, a critical feature in fields demanding high credibility, like research and journalism.
Implementing RAG in Artificial Intelligence involves integrating a language model with a retrieval system that pulls relevant data from external knowledge bases, generating contextually accurate, fact-based responses. Unlike semantic search, which retrieves existing information based on query intent, RAG actively creates responses grounded in sourced data.
RAG in Action: Real-World Applications
The advent of Retrieval-Augmented Generation (RAG) has opened up a plethora of opportunities for enhancing artificial intelligence applications in the real world. By combining the generative capabilities of models like the Generative Pre-trained Transformer (GPT) with the ability to dynamically pull in information from various databases, RAG is transforming industries and how they interact with AI. Here, we explore some compelling real-world applications of RAG across different sectors.
Customer Service Enhancement
In the realm of customer service, RAG-powered chatbots and virtual assistants are making significant strides. Unlike traditional bots that rely on a static set of responses, RAG-enabled solutions can fetch and incorporate the latest information from external sources in real-time. This capability ensures that customers receive up-to-date, accurate, and personalized responses to their queries, greatly enhancing the customer service experience.
Content Creation and Summarization
For content creators, RAG offers the tools to generate rich, informative, and current content. Journalists, researchers, and marketers can use RAG to quickly produce summaries of recent developments, reports, and articles. By grounding content generation in the most recent sources, RAG ensures the output is both relevant and credible, a crucial aspect in today's fast-paced information landscape.
Personalized Education and Learning
Education technology is another field reaping the benefits of RAG in chatbot development. Tailored learning experiences are crafted by dynamically sourcing and integrating information based on a learner's progress, interests, and areas of difficulty. This personalized approach, powered by RAG, not only makes learning more engaging but also more effective, catering directly to the individual's learning style and needs.
Medical Research and Healthcare
In healthcare, RAG can assist medical professionals by providing the latest research findings, treatment options, and clinical data relevant to a patient's specific condition. This application of RAG in medical research and healthcare not only saves valuable time but also ensures that patient care is informed by the most current and comprehensive information available.
Financial Services
Financial analysts can leverage RAG to stay ahead of market trends and developments. By automating the retrieval of the latest financial reports, market analysis, and news, RAG allows for the generation of insights and forecasts that are deeply informed by the latest data. This real-time analysis can provide businesses and investors with a competitive edge in fast-moving markets.
These applications of RAG showcase its versatility and potential to revolutionize how businesses and services operate. By harnessing the power of up-to-date information and Generative AI, RAG is setting a new standard for intelligence, efficiency, and personalization across diverse sectors.
Step-by-Step Guide to Implementing RAG with LangChain
Step 1: Set Up Python Environment
Create a virtual environment to isolate your project dependencies.
# Create virtual environment
$ python -m venv myenv
# Activate the virtual environment
# On Windows
$ myenv\Scripts\activate
# On macOS/Linux
$ source myenv/bin/activate
Step 2: Install Required Libraries
Ensure you have the necessary Python libraries installed. You can install them using pip:
$ pip install streamlit pypdf langchain langchain_openai langchain_community faiss-cpu
Step 3: Import Libraries
Start by importing the necessary libraries at the beginning of your script:
import os
import pathlib
import streamlit as st
from pypdf import PdfReader
from tempfile import NamedTemporaryFile
from langchain.docstore.document import Document
from langchain_core.messages import HumanMessage, SystemMessage
from langchain_openai import ChatOpenAI
from langchain.chains.question_answering import load_qa_chain
from langchain_openai import OpenAIEmbeddings
from langchain_community.vectorstores import FAISS
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain_community.document_loaders.csv_loader import CSVLoader
Step 4: Define Utility Functions
Define the functions that will handle file processing and conversion:
convert_to_json
: Converts document content to JSON format.prepare_files
: Prepares files for processing by extracting their content.handle_pdf_file
: Extracts text content from PDF files.handle_csv_file
: Extracts content from CSV files.
def convert_to_json(document_content):
messages = [
SystemMessage(content="System message"),
HumanMessage(content=document_content)
]
answer = chat.invoke(messages)
return answer.content
def prepare_files(files):
document_content = ""
for file in files:
if file.type == 'application/pdf':
page_contents = handle_pdf_file(file)
elif file.type == 'text/csv':
page_contents = handle_csv_file(file)
else:
st.write('File type is not supported!')
document_content += "".join(page_contents)
return document_content
def handle_pdf_file(pdf_file):
document_content = ''
with pdf_file as file:
pdf_reader = PdfReader(file)
page_contents = []
for page in pdf_reader.pages:
page_contents.append(page.extract_text())
document_content += "\n".join(page_contents)
return document_content
def handle_csv_file(csv_file):
with csv_file as file:
uploaded_file = file.read()
with NamedTemporaryFile(dir='.', suffix='.csv') as f:
f.write(uploaded_file)
f.flush()
loader = CSVLoader(file_path=f.name)
document_content = "".join([doc.page_content for doc in loader.load()])
return document_content
Step 5: Configure Streamlit Interface
Set up the Streamlit page configuration and interface elements for uploading files, entering the OpenAI API key, and inputting the query:
st.set_page_config(page_title='AI PDF Chatbot', page_icon=None, layout="centered", initial_sidebar_state="auto", menu_items=None)
st.title("PDF Chatbot")
files = st.file_uploader("Upload PDF and CSV files:", accept_multiple_files=True, type=["csv", "pdf"])
openai_key = st.text_input("Enter your OpenAI API key:")
if openai_key:
os.environ["OPENAI_API_KEY"] = openai_key
chat = ChatOpenAI(model_name='gpt-4', temperature=0)
embeddings = OpenAIEmbeddings()
query = st.text_input("Enter your query for the document data:")
text_splitter = RecursiveCharacterTextSplitter(chunk_size=10000, chunk_overlap=1000)
Step 6: Implement Query Handling Logic
Add the logic to handle the query when the "Get Answer to Query" button is clicked. This includes preparing the files, splitting the text, searching for similar chunks, and running the QA chain.
if st.button("Get Answer to Query"):
if files and openai_key and query:
pdb.set_trace()
document_content = prepare_files(files)
chunks = text_splitter.split_text(document_content)
db = FAISS.from_texts(chunks, embeddings)
chain = load_qa_chain(chat, chain_type="stuff", verbose=True)
docs = db.similarity_search(query)
print("docsearch", docs)
response = chain.run(input_documents=docs, question=query)
st.write("Query Answer:")
st.write(response)
else:
st.warning("Please upload PDF and CSV files, enter your OpenAI API key, and enter your query")
Running the Application
Save your script as app.py
and run it using the following command:
$ streamlit run app.py
This will start the Streamlit application, allowing you to upload PDF and CSV files, enter your OpenAI API key, and input a query to get answers from the document content.
Challenges and Solutions in RAG Deployment
As revolutionary as Retrieval-Augmented Generation (RAG) is for enhancing Large Language Models (LLMs) with the latest, most relevant information, deploying it comes with its own set of challenges. From data management to maintaining model accuracy, each hurdle requires strategic solutions to leverage RAG's full potential. This section examines common obstacles encountered during RAG deployment and proposes practical solutions.
Data Quality and Accessibility
One of the primary challenges in RAG deployment is ensuring the quality and accessibility of data. RAG systems rely on external databases to retrieve information, making the accuracy and relevance of this data crucial. Poor data quality can lead to misinformation and reduce the efficacy of the generative models.
Solution: Implement robust data validation and curation processes. Utilize automated tools to regularly check the integrity and relevance of the data. Additionally, placing a layer of human oversight can help identify and rectify data inaccuracies that automated systems might miss.
Integrating Diverse Data Sources
Another challenge is the integration of heterogeneous data sources. RAG systems may need to pull information from varied formats and repositories, which can complicate data retrieval and processing.
Solution: Standardize data formats as much as possible and employ middleware or adapters that can translate between different data schemas. This approach can streamline data integration, making it more efficient for RAG systems to retrieve and utilize the necessary information.
Scalability and Performance
As the volume of data grows, maintaining the scalability and performance of RAG systems becomes increasingly challenging. High query volumes can lead to latency issues, affecting the user experience.
Solution: Optimize your infrastructure for scalability from the outset. Consider cloud-based solutions that offer elasticity based on demand. Additionally, implementing caching strategies for frequently requested information can significantly reduce retrieval times.
Model Fine-tuning and Updating
Keeping the underlying LLMs up-to-date with the latest information without frequent retraining is a challenge. The dynamic nature of information means that RAG systems must continuously adapt to maintain accuracy.
Solution: Employ incremental learning techniques where the model can learn from new data without the need for complete retraining. This approach enslures that the model remains current with minimal computational overhead.
Ensuring Privacy and Security
When RAG systems access external data sources, they must navigate privacy and security concerns. Ensuring that sensitive information is not inadvertently retrieved or exposed is paramount.
Solution: Implement strict access controls and data governance policies. Use encryption for data in transit and at rest. Additionally, anonymize or redact sensitive information from the data used by RAG systems to mitigate privacy concerns.
Summary
RAG is transforming the landscape of AI by enhancing the capabilities of large language models. As a leading chatbot development company, Bluebash leverages RAG to create intelligent solutions that provide accurate, personalized, and contextually relevant responses, ultimately improving user experiences across various industries.
FAQ's
- What is LLM and RAG?
LLM stands for Large Language Model, which refers to a type of AI model that is trained on vast amounts of text data to understand and generate human-like text. RAG stands for Retrieval-Augmented Generation, a technique that combines the generative capabilities of LLMs with information retrieval systems to enhance the relevance and accuracy of generated text.
- What is the full meaning of RAG?
RAG stands for Retrieval-Augmented Generation.
- What is RAG used for?
RAG is used to improve the quality of text generation by integrating retrieval mechanisms. It allows models to fetch relevant information from a knowledge base or database, which can then be used to generate more accurate and contextually relevant responses.
- What is Retrieval-Augmented Generation?
Retrieval-Augmented Generation (RAG) is a technique in AI where a generative model, like an LLM, is combined with a retrieval system. This system retrieves relevant documents or data points from an external database or knowledge base, and the generative model uses this information to produce more accurate and contextually appropriate responses.
- What is RAG in generative AI?
In generative AI, RAG refers to the combination of retrieval systems and generative models to enhance the quality of generated outputs. By accessing and utilizing relevant external information, generative models can produce more informed and precise responses.
- What is the difference between RAG and LLM?
LLM (Large Language Model) refers to the model itself, which is capable of generating text based on learned patterns from a large corpus of data. RAG (Retrieval-Augmented Generation) is a technique that enhances an LLM's performance by integrating it with a retrieval system to fetch relevant information, which is then used by the LLM to generate more accurate and contextually appropriate responses.
- How to improve retrieval in augmented generation?
Improving retrieval in augmented generation can be achieved by:
- Enhancing the quality and relevance of the knowledge base or database used for retrieval.
- Utilizing advanced retrieval algorithms that better understand and match queries with relevant information.
- Continuously updating the retrieval system with new data to ensure the most current information is available.
- What are retrieval techniques?
Retrieval techniques are methods used to find and fetch relevant information from a database or knowledge base. Common techniques include keyword matching, semantic search, vector similarity search, and hybrid approaches that combine multiple methods to improve accuracy and relevance.
- What are the two types of retrieval?
The two main types of retrieval are:
- Exact Match Retrieval, which finds information based on exact keyword matches.
- Semantic Retrieval, which uses natural language processing to understand the meaning behind queries and fetch information that is contextually relevant, even if the exact keywords are not present.
- What is the difference between GenAI and RAG?
GenAI, or Generative AI, refers to AI models that can generate new content, such as text, images, or music, based on learned patterns from training data. RAG, or Retrieval-Augmented Generation, specifically enhances generative AI by incorporating retrieval systems to access relevant external information, thereby improving the relevance and accuracy of the generated content.