Beginner's Guide to Install Advanced PyTorch Applications
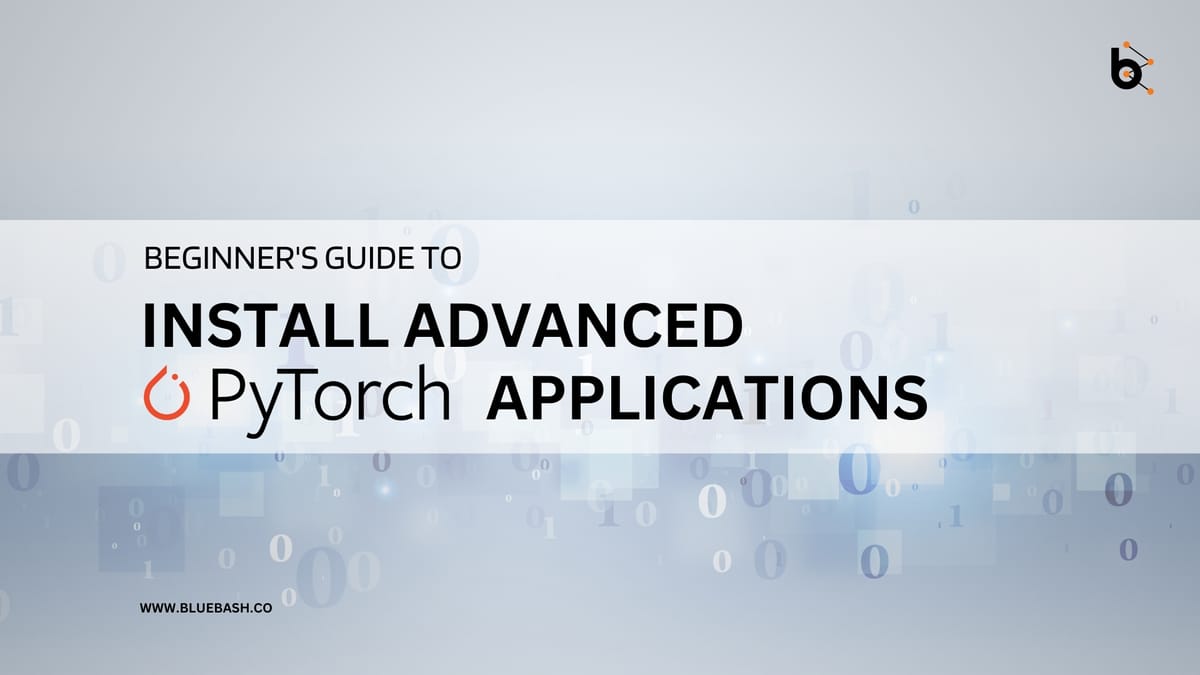
In the rapidly evolving landscape of artificial intelligence and machine learning, one framework has emerged as a particularly powerful and user-friendly tool for developers and researchers alike: PyTorch. Developed by the AI team at Facebook, PyTorch has gained immense popularity for its ease of use, flexibility, and dynamic computational graph. In this introduction, we will explore what makes PyTorch stand out, its ecosystem, and how you can get started with this versatile tool.
What is PyTorch?
At its core, PyTorch is an open-source machine learning library based on the Torch library, extensively used for applications such as computer vision and natural language processing. What sets PyTorch apart is its imperative programming model, which allows software developers to change the behavior of their program on the fly and use natural Python syntax, making it incredibly intuitive, especially for beginners in the field of deep learning.
Why Choose PyTorch?
One may wonder, with several frameworks available, why PyTorch has seen such a rapid adoption rate. The answer lies in its simplicity and the power it offers to developers. Unlike other frameworks that may require learning new paradigms or languages, PyTorch leverages Python's simplicity, making it accessible to a wide audience. Furthermore, its dynamic computation graph enables more natural debugging and a flexible approach to model building, which is particularly beneficial in research and development settings.
Another aspect where PyTorch shines is its documentation and community support. With extensive resources, tutorials, and a vibrant community on platforms like GitHub, new users can quickly find answers to their queries and delve deeper into advanced topics.
Setting Up PyTorch: A Step-by-Step Guide
Preparing Your Environment
Before diving into the world of deep learning with PyTorch, it's crucial to set up your development environment correctly. This guide will walk you through the process, ensuring that you have all the necessary tools and dependencies installed on your machine.
Install Anaconda or Miniconda
Firstly, having a Python environment manager like Anaconda or Miniconda is highly recommended. These tools simplify package management and deployment, making it easier to manage multiple Python environments with potentially conflicting dependencies. Install Anaconda (or Miniconda for a lighter version) by following the instructions on their respective websites.
Setting Up CUDA for GPU Support
If you're planning to leverage the power of your NVIDIA GPU for PyTorch projects, installing CUDA is a must. CUDA allows PyTorch to access the GPU's parallel computing capabilities, significantly speeding up training times for deep learning models. Visit the NVIDIA website to download and install the appropriate version of CUDA for your GPU. Remember, compatibility between your NVIDIA drivers, CUDA, and PyTorch is key, so ensure you're installing the correct versions.
# Verify CUDA installation
nvcc --version
Installing PyTorch
With your environment and CUDA ready, installing PyTorch is straightforward. Open your terminal or Anaconda Prompt and use the following command to install PyTorch along with torchvision and torchaudio, which are libraries that provide useful utilities for vision and audio tasks respectively.
# For Conda users
conda install pytorch torchvision torchaudio cudatoolkit=11.3 -c pytorch
# For pip users
pip install torch torchvision torchaudio
Note: Replace "cudatoolkit=11.3" with the version compatible with your CUDA installation.
Verifying the Installation
After installation, it's a good practice to verify that PyTorch is correctly installed and can access your GPU (if available). Run the following Python code in your terminal or Python environment:
import torch
print(torch.__version__)
print('CUDA available:', torch.cuda.is_available())
print('CUDA version:', torch.version.cuda)
If everything is set up correctly, you should see the PyTorch version printed, along with a confirmation that CUDA is available and its version.
Understanding PyTorch's Dynamic Computational Graph
What Sets PyTorch Apart: Dynamic vs. Static Graphs
In the realm of deep learning frameworks, PyTorch is distinguished for its dynamic computational graph, also known as a define-by-run paradigm. Unlike other frameworks like TensorFlow, which employ a static graph structure, PyTorch constructs the computation graph on the fly. This means that the graph is built at each point of execution, allowing for more flexibility and interactive debugging during development.
Dynamic Computation Graphs Explained
A computational graph is a representation of data and operations in the form of nodes and edges. In PyTorch, each operation on tensors becomes a node in the graph, dynamically reflecting the execution of your program. This approach contrasts with static graphs, where the graph is defined and compiled before execution, possibly making it less intuitive to modify or debug.
import torch
# Example of dynamic graph construction
x = torch.tensor(1., requires_grad=True)
y = torch.tensor(2., requires_grad=True)
z = x**2 + y**3
z.backward()
print(f"x.grad: {x.grad}, y.grad: {y.grad}")
This snippet demonstrates how straightforward it is to perform operations and automatically compute gradients in PyTorch. The computation graph is built when `z.backward()` is called, calculating the gradients of `z` with respect to `x` and `y`, showcasing the dynamic nature of the graph.
Advantages of Dynamic Graphs
- Flexibility: Since the graph is created at runtime, you can change the behavior of your model dynamically based on the data. This is particularly useful for models that are conditional or vary in size, such as with varying input lengths in sequences.
- Debugging: Debugging is more intuitive as the graph construction is intertwined with Python execution. This means you can use standard Python debugging tools and practices to debug your model.
Iterative Development: The define-by-run nature of PyTorch allows for an interactive and experimental approach to model building, making it easier to refine and adjust models on the go.
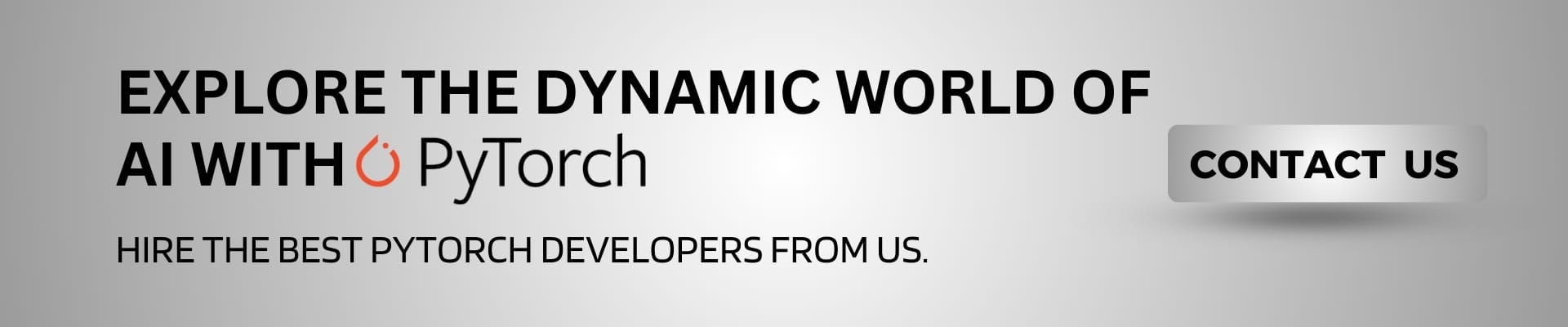
Building Your First Neural Network in PyTorch
Embarking on the journey of neural network development in PyTorch begins with understanding the basic building blocks. A neural network typically consists of layers of neurons that process input data, learn from it, and make predictions or classifications. PyTorch simplifies this process with its comprehensive set of tools and libraries.
Defining a Simple Neural Network
Let's start by defining a simple neural network in PyTorch. This example will illustrate how to construct a network consisting of fully connected layers, also known as linear layers, which form the backbone of many deep learning models.
import torch
import torch.nn as nn
import torch.nn.functional as F
class SimpleNet(nn.Module):
def __init__(self):
super(SimpleNet, self).__init__()
# Define layers
self.fc1 = nn.Linear(in_features=784, out_features=128)
self.fc2 = nn.Linear(in_features=128, out_features=64)
self.output = nn.Linear(in_features=64, out_features=10)
def forward(self, x):
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = self.output(x)
return x
This code snippet defines a neural network with two hidden layers and an output layer. The `forward` method specifies how the data flows through the network, with ReLU activations applied to the hidden layers.
Training the Network
With our network defined, the next step is training it on data. Training involves feeding data through the network, calculating the loss (i.e., how far off the predictions are from the actual values), and adjusting the weights of the network in a way that minimizes this loss over time.
# Assuming `data_loader` is a PyTorch DataLoader object containing our training data
optimizer = torch.optim.Adam(SimpleNet.parameters(), lr=0.001)
criterion = nn.CrossEntropyLoss()
for epoch in range(epochs):
for inputs, labels in data_loader:
outputs = SimpleNet(inputs)
loss = criterion(outputs, labels)
optimizer.zero_grad()
loss.backward()
optimizer.step()
print(f"Epoch {epoch+1}, Loss: {loss.item()}")
This snippet demonstrates a basic training loop, where the model's predictions are compared against actual labels using cross-entropy loss, and the Adam optimizer is used to update the network's weights based on the computed gradients.
Evaluating the Network
After training, evaluating the network on unseen data is crucial to understanding its performance. This typically involves calculating accuracy or other metrics on a test dataset.
correct = 0
total = 0
with torch.no_grad():
for inputs, labels in test_loader:
outputs = SimpleNet(inputs)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print(f"Accuracy: {100 * correct / total}%")
This code calculates the accuracy of the model by comparing the predicted labels with the actual labels in the test dataset.
Leveraging Pre-trained Models for Quick Wins
The Power of Pre-trained Models
One of the most compelling features of PyTorch is its extensive collection of pre-trained models. These models, which have been trained on vast datasets, offer a shortcut for developers and researchers to achieve state-of-the-art results without the heavy lifting of training models from scratch. This approach not only saves time but also computational resources, making advanced machine learning more accessible to everyone.
Exploring PyTorch Model Zoo
PyTorch provides access to a "model zoo," a repository of pre-trained models that can be easily downloaded and integrated into your projects. These models cover a wide range of applications, from image classification and object detection to natural language processing and beyond. Leveraging these models can significantly accelerate the development of AI applications.
Code Snippet: Using a Pre-trained Model
import torch
import torchvision.models as models
# Load a pre-trained ResNet model
resnet = models.resnet18(pretrained=True)
# Prepare your image (to be added)
# image = ...
# Model in evaluation mode
resnet.eval()
# Perform inference
# predictions = resnet(image)
This simple example demonstrates how to load a pre-trained ResNet model from PyTorch's torchvision module. Note that you'll need to preprocess your image before feeding it into the model for inference.
Why Use Pre-trained Models?
- Efficiency: Training models, especially deep learning models, can be time-consuming and resource-intensive. Pre-trained models offer a way to bypass this by providing a ready-made solution that can be fine-tuned for specific tasks.
- Accessibility: They democratize AI by making cutting-edge models accessible to developers and researchers without the need for extensive computational resources.
- Transfer Learning: Pre-trained models are an excellent basis for transfer learning, where the knowledge from one task is leveraged to improve performance on a different but related task.
Customizing Pre-trained Models
While pre-trained models offer a great starting point, they often need to be customized or fine-tuned to fit the specific needs of your project. PyTorch's flexible architecture makes this process straightforward. By adjusting the final layers of the model or tweaking the training parameters, you can adapt pre-trained models to new tasks with minimal effort.
# Example of fine-tuning a pre-trained model in PyTorch
resnet.fc = torch.nn.Linear(resnet.fc.in_features, 100) # Adjusting for 100 output classes
# Fine-tuning process (to be added)
# ...
In this example, we modify the fully connected layer of the ResNet model to output 100 classes instead of its original setting. This is a common step in adapting a model for a different number of output classes.
Advanced PyTorch: Custom Layers and Operations
Expanding Your PyTorch Toolkit
As you delve deeper into the world of deep learning with PyTorch, you might find yourself in need of more specialized tools than what the standard library offers. This is where the power of PyTorch really shines through - its flexibility allows you to create custom layers and operations that can tailor the framework to your specific project needs. In this section, we explore how to extend PyTorch's capabilities by implementing custom layers and operations, a technique that can significantly enhance the functionality and efficiency of your neural networks.
Creating Custom Layers in PyTorch
While PyTorch's extensive collection of pre-built layers covers a wide range of deep learning applications, there might be instances where you need a layer with functionality that's not available out of the box. Fortunately, creating custom layers in PyTorch is straightforward, thanks to its dynamic nature and Pythonic design.
import torch.nn as nn
import torch
class CustomLinearLayer(nn.Module):
def __init__(self, input_features, output_features):
super(CustomLinearLayer, self).__init__()
self.weights = nn.Parameter(torch.randn(input_features, output_features))
self.bias = nn.Parameter(torch.randn(output_features))
def forward(self, x):
return torch.matmul(x, self.weights) + self.bias
This code snippet showcases how to define a simple custom linear layer. By subclassing nn.Module
and defining a forward
method, you can create layers that behave just like any other PyTorch layer.
Implementing Custom Operations
There are times when you might need to go beyond the provided operations and define your own, whether for performance optimization or to introduce novel functionality into your models. PyTorch's flexibility makes implementing custom operations a seamless experience.
class CustomReLU(nn.Module):
def __init__(self):
super(CustomReLU, self).__init__()
def forward(self, x):
return torch.max(torch.tensor(0.0), x)
This example demonstrates how to implement a custom ReLU (Rectified Linear Unit) operation. While PyTorch already has a ReLU function, creating your own can be a great exercise in understanding how operations work under the hood.
Integrating Custom Components
Once you've created your custom layers and operations, integrating them into your models is as easy as using any standard component. This allows you to experiment and iterate rapidly, a core strength of PyTorch.
model = nn.Sequential(
CustomLinearLayer(784, 256),
CustomReLU(),
nn.Linear(256, 10)
)
In this model, we incorporate our custom linear layer and ReLU operation alongside standard PyTorch layers, showcasing the framework's modularity and ease of use.
FAQ's
- What is PyTorch used for?
PyTorch is a popular open-source machine learning framework, particularly strong in deep learning. It's used for building and training neural networks for tasks like image recognition, natural language processing, and recommender systems. Additionally, it's utilized in research due to its flexibility and ease of debugging, as well as in production deployment with tools for scaling to large datasets and hardware. - Is PyTorch better than TensorFlow?
There's no definitive "better". Here's a breakdown: PyTorch is more user-friendly, good for rapid prototyping and research. TensorFlow, on the other hand, is more established with wider industry adoption and a larger community. They both achieve similar results, so the choice often depends on your project and preferences. - Is PyTorch same as Python?
No. PyTorch is a library written in C++ for use with Python. It provides deep learning tools and functionalities on top of the Python language. - Is PyTorch written in C or C++?
PyTorch's core is written in C++ for performance, but it has a Python interface for user-friendliness. - Can I learn PyTorch without Python?
No. Since PyTorch uses Python, basic Python knowledge is necessary to effectively use PyTorch libraries and functionalities. - Is PyTorch similar to Keras?
Yes, both are deep learning frameworks. Keras can actually run on top of PyTorch or TensorFlow, providing a higher-level interface. - Does OpenAI use PyTorch or TensorFlow?
OpenAI uses both. They don't publicly disclose specifics, but research papers suggest they leverage the advantages of both frameworks for different tasks. - Is ChatGPT built on PyTorch?
OpenAI hasn't revealed the specific underlying technology of ChatGPT. While PyTorch is a possibility, it could also be TensorFlow or a custom solution. - Is PyTorch used for AI?
Yes! PyTorch is a powerful tool for developing artificial intelligence, specifically in the area of deep learning and neural networks. - Which big companies use PyTorch?
Many leading companies leverage PyTorch, including Facebook (originator), Meta, Uber, Twitter, and Tesla.